Project Brief:
Create an Internet-connected device using at least 2 sensors. The choice of sensors is up to you, it can be anything from a button or a potentiometer to .... This device will collect data in your house for a week. Consider where it's going to be installed, how it's going to be powered. Are there any mechanical components you need to add to translate action to your sensors (levers, pulleys, springs, etc)? How often will you be saving this data to the database - consider both the limitations (no more than 30 data points per feed on AdafruitIO, for example) and the necessity (do you need repeated values or only the changed ones.)
Create an Internet-connected device using at least 2 sensors. The choice of sensors is up to you, it can be anything from a button or a potentiometer to .... This device will collect data in your house for a week. Consider where it's going to be installed, how it's going to be powered. Are there any mechanical components you need to add to translate action to your sensors (levers, pulleys, springs, etc)? How often will you be saving this data to the database - consider both the limitations (no more than 30 data points per feed on AdafruitIO, for example) and the necessity (do you need repeated values or only the changed ones.)
Project Goal:
Creating an IoT device that collects light intensity and temperature data and visualizes it dynamically.
Visualization Concept:
Light intensity controls firework size.
Temperature controls firework color.
Components and Setup:
ESP32 (microcontroller).
Light sensor (photoresistor).
Temperature sensor (TMP36).
Arduino Code:
#include "config.h"
// Define the sensors
#define TEMP_SENSOR_PIN A2 // Temperature sensor
#define PHOTOCELL_PIN A3 // Photoresistor remains the same
// Feeds for Adafruit IO
AdafruitIO_Feed *tempFeed = io.feed("temperature-feed");
AdafruitIO_Feed *photoFeed = io.feed("photo-feed");
void setup() {
// Initialize Serial for debugging
Serial.begin(9600);
while (!Serial){}
Serial.println("Starting...");
Serial.print("Connecting to Adafruit IO");
io.connect();
// Wait for a connection to Adafruit IO
while (io.status() < AIO_CONNECTED) {
Serial.print(".");
delay(500);
}
Serial.println();
Serial.println(io.statusText());
}
void loop() {
io.run(); // Required to maintain Adafruit IO connection
// Read sensor values
int rawTempVal = analogRead(TEMP_SENSOR_PIN); // Read raw analog value from the temperature sensor
float tempC = (rawTempVal * 3.3 / 4096.0 - 0.35) * 100.0; // Convert to Celsius
float photoVal = analogRead(PHOTOCELL_PIN); // Read photoresistor value
// Send temperature data to Adafruit IO
Serial.print("Sending temperature: ");
Serial.print(tempC);
Serial.println(" °C");
tempFeed->save(tempC); // Send temperature to Adafruit IO
// Send photoresistor data to Adafruit IO
Serial.print("Sending photoresistor: ");
Serial.println(photoVal);
photoFeed->save(photoVal); // Send light value to Adafruit IO
delay(5000); // Wait for 5 seconds before sending the next values
}
Data Collection Process:
I placed the device near my house plant. Where there are windows for light.
The data was received once every 5 seconds.
Device placed near house plant
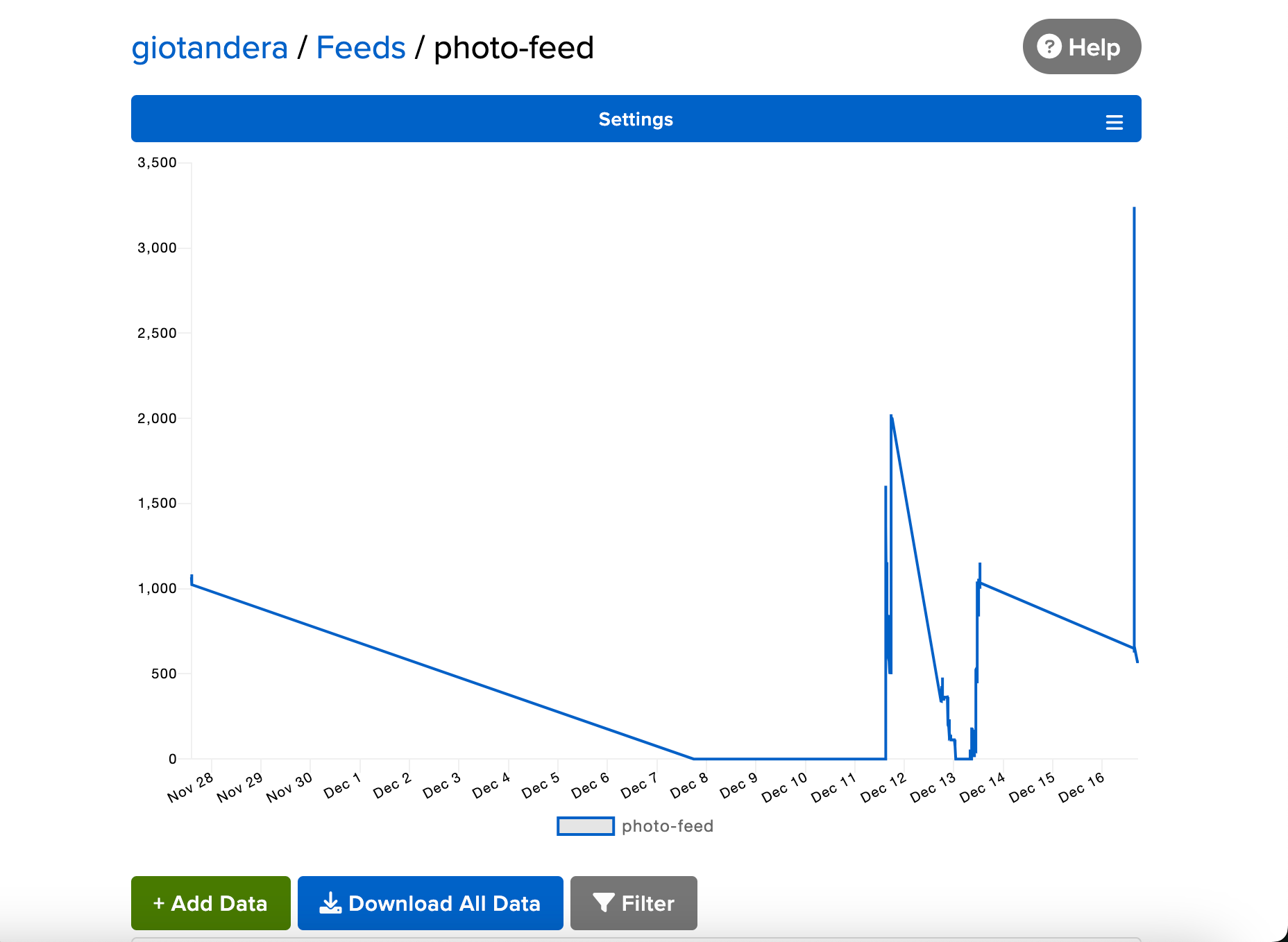
Adafruit Photo Feed
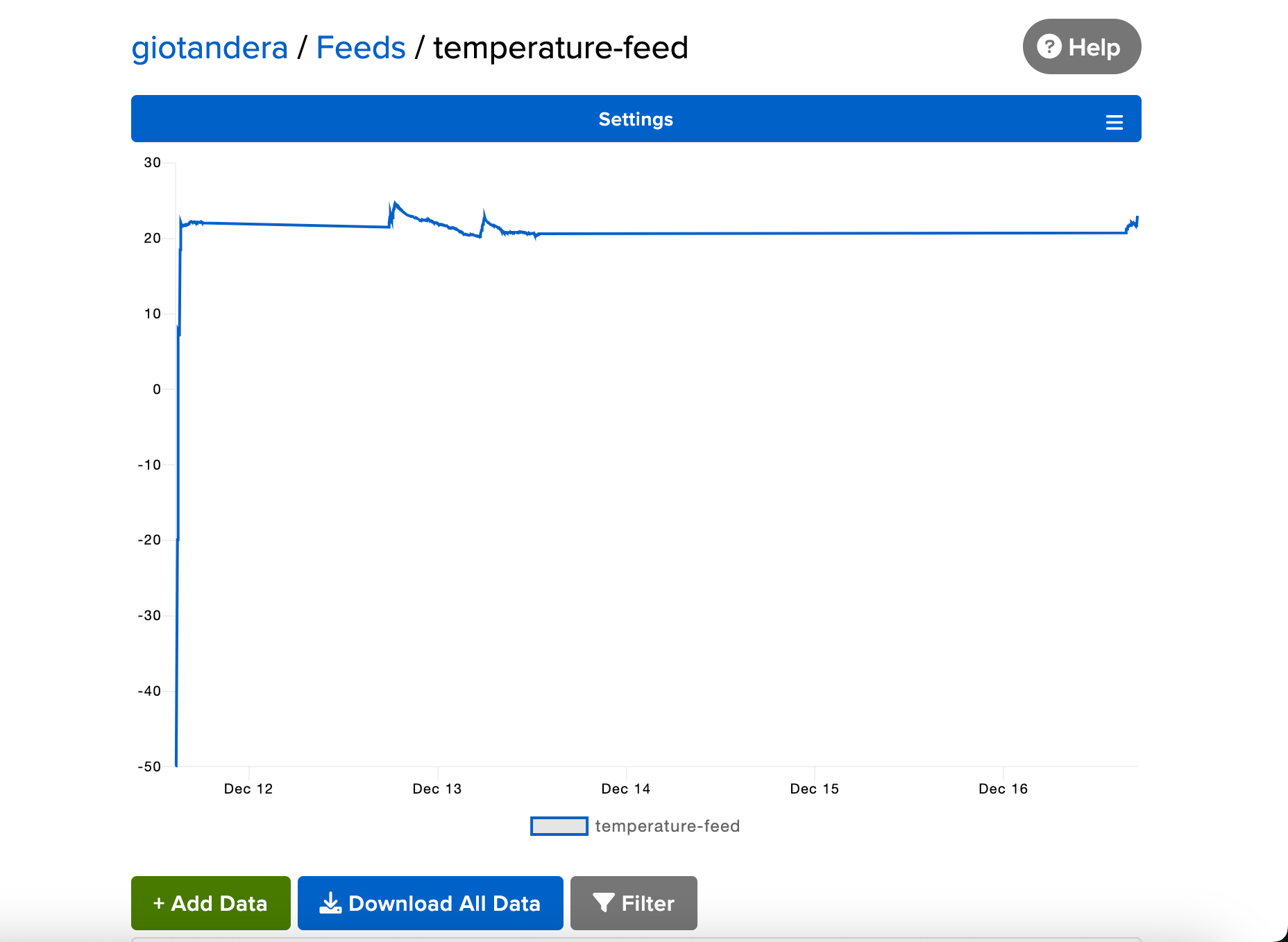